It seems to be black magic but actually it is not, there is an abstraction layer implemented behind the scenes which actually acts as a facade between the external content source and Tridion.
Let's talk about the abstraction layer mentioned above, well that abstraction layer is implemented using the Microsoft Add Ins framework (for developers System.AddIn), so by having an Add Ins framework in place it is really easy to add and remove ECLs without needing to perform major changes.
OK, so let's provide some details about what is the Microsoft Add Ins Framework, I believe it is worth to understand it before going further on ECL implementation.
Microsoft Add Ins Framework with an ECL perspective
As shown in the following picture this framework is composed by seven pieces that work together in order to provide a flexible plug and play mechanism.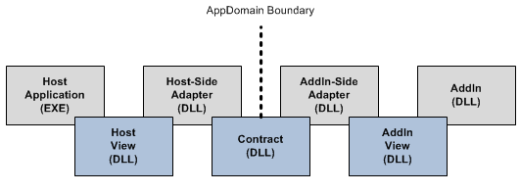
Basically what we need to do is to define a contract (Inteface) which will define the methods and functionality that should be met for both Host and AddIn, as you may noticed the Host is the Tridion Content Manager Service Host - Window Service which already implements the Host View and the Host-Side Adapter.
The Host View is a server side version of the contract but in most of the cases is not the same that is the reason why we need a Host-Side Adapter that acts as a translator between the Host View and the Contract.
The same thing happens in the AddIn (ECL Provider) side. In ECL for Tridion 2013 all this functionality is already in place, what we need to do is actually to implement the AddIn View so that we can have a working AddIn-Side Adapter.
ECL for Tridion 2013 Details
An ECL (AddIn) is composed by four principal pieces: Content Library, Content Library Context, Content Library Item and Content Library List Item (AddIn View).Content Library
This is actually the entry point for the ECL execution, it is responsible to create the Content Library Context and to initialize the AddIn. In order to create a Content Library the class must implement the IContentLibrary interface.Content Library Context
This could be considered as the business/data logic for the ECL, it is responsible to connect to the external content source and to retrieve the external content. This piece will use data coming from the Content Library and also will use the Content Library Items and List Items. In order to create a Content Library Context the class must implement the IContentLibraryContext.Content Library Item
This is a DTO that maps with an external content item. This one implements the IContentLibraryMultimediaItem by implementing a set of properties that will provide access to information and metadata available in the external content item.Content Library List Item
This is a DTO that maps with an external content folder/directory. This one implements the IContentLibraryListItem by implementing a set of properties that will provide access to information and metadata available in the external content folder.Installing and Configuring an ECL in Tridion 2013
Installing an ECL
By default ECLs are installed at "C:\ProgramData\SDL\SDL Tridion\External Content Library\AddInPipeline\AddIns"To install a new AddIn just create a new folder inside the AddIns folder and copy the DLL containing your AddIn there.
Configuring an ECL
ECL is configured in configuration file "ExternalContentLibrary.xml". This file is located at [TRIDION_HOME]\config folder.In order to configure a new ECL, it is needed to add a new Mount Point in the configuration.
<MountPoint type="FileSystemProvider" version="*" id="filesystem" rootItemName="filesystem">
<StubFolders>
<StubFolder id="tcm:4-30-2" />
</StubFolders>
<PrivilegedUserName>T2013\Administrator</PrivilegedUserName>
<FileSystemRoot>C:\ECL\FileSystem</FileSystemRoot>
</MountPoint>
- type attribute: This one should match with the AddIn name installed above
- id: Unique identifier for the Mount Point.
- rootItemName: The CME will use this value to create the root node in the Tree Explorer.
- StubFolder: Folder where the stub components will be created. A stub component is a link between an external content item and Tridion.
- PrivilegedUserName: User with system admin privileges.
- Any custom configuration element, in this case I am adding a new one called FileSystemRoot.
Developing an ECL in Tridion 2013
I am not providing full source code since it is very long and not suitable for a blog. I can share it for all the interested ones :-).Creating a Content Library
[AddIn("FileSystemProvider",
Description="File System External Content Library Provider",
Publisher="Eric Huiza",
Version="1.0.0.0")]
public class FileSystemEclProvider : IContentLibrary {
...
public IContentLibraryContext CreateContext(IEclSession session) {
return new FileSystemEclProviderContext();
}
public void Initialize(
string mountPointId,
string configurationXmlElement,
IHostServices hostServices) {
MountPointId = mountPointId;
HostServices = hostServices;
XElement configuration = XElement.Parse(configurationXmlElement);
Root = configuration.Element(
XNamespace.Get("http://www.sdltridion.com/ExternalContentLibrary/Configuration")
+ "FileSystemRoot").Value;
}
public IList<IDisplayType> DisplayTypes {
get {
return new List<IDisplayType> {
HostServices.CreateDisplayType("folder", "Folder", EclItemTypes.Folder),
HostServices.CreateDisplayType("img", "Image", EclItemTypes.File)
};
}
}
public byte[] GetIconImage(string theme, string iconIdentifier, int iconSize) {
return null;
}
public void Dispose() {
}
}
Creating a Content Library Context
public class FileSystemEclProviderContext : IContentLibraryContext {. . .
}
Creating a Content Library Item
public class File : IContentLibraryMultimediaItem {. . .
}
Creating a Content Library List Item
public class Folder : IContentLibraryListItem, IContentLibraryItem {. . .
}
The result will be like this. Please note that at this moment ECL for Tridion 2013 just supports Multimedia Items.